So i have been trying to use some Revit API to extract ElementIds from elements. Here’s the code:
import clr
clr.AddReference(‘ProtoGeometry’)
from Autodesk.DesignScript.Geometry import *
# Import RevitAPI
clr.AddReference(“RevitAPI”)
import Autodesk
from Autodesk.Revit.DB import *
# Import ToDSType(bool) extension method
clr.AddReference(“RevitNodes”)
import Revit
clr.ImportExtensions(Revit.Elements)
#The inputs to this node will be stored as a list in the IN variable.
dataEnteringNode = IN
elements = []
for i in IN[0]:
elements.append(UnwrapElement(i))
elementIds, idString, guid = [], [], []
for i in elements:
elementIds = i.Id.ToDSType(true)
idString = i.Id.ToString().ToDSType(true)
guid = i.UniqueId.ToDSType(true)
#Assign your output to the OUT variable
OUT = elementIds, idString, guid
Here’s the error that i keep getting.
Warning: IronPythonEvaluator.EvaluateIronPythonScript operation failed.
‘ElementId’ object has no attribute ‘ToDSType’
Any ideas?
Thank you,
Konrad
hi konrad, i published a package called guid tools
it consists of three nodes
-
extract guids
-
extract Ids
-
extract both
hope that helps and the code works
peter
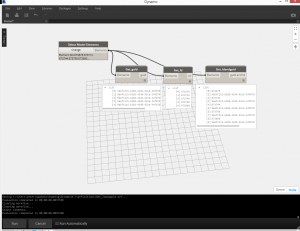
Hey Konrad,
An ElementId is not an Element, so the extension method ToDSType() is not present. You can return the integer just by doing:
eleId.IntegerValue
~Peter
Peter B,
I see now. Only do i need to wrap it if i am creating an actual Element like a point, curve, view or annotation tag but not when I am just pulling out their properties. This makes more sense now. Thank you,
Peter K,
Thank you for the link. I didn’t see your nodes before, but then again I wrapped it all up into one Python node called Element Id that can get you anything you need. Here’s the code:
import clr
clr.AddReference(‘ProtoGeometry’)
from Autodesk.DesignScript.Geometry import *
Import RevitAPI
clr.AddReference(‘RevitAPI’)
import Autodesk
#The inputs to this node will be stored as a list in the IN variable.
dataEnteringNode = IN
elements = []
for i in IN[0]:
elements.append(UnwrapElement(i))
elementIds, idString, guid = [], [], []
for i in elements:
elementIds.append(i.Id)
idString.append(i.Id.ToString())
guid.append(i.UniqueId)
#Assign your output to the OUT variable
OUT = elementIds, idString, guid
Thank you,